目录
介绍
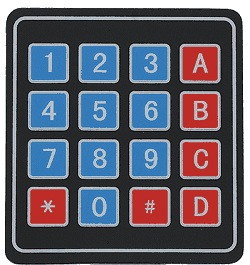
键盘用作输入设备,用于读取用户按下的键并进行处理。
4×4键盘由4行和4列组成。开关位于行和列之间。按键在相应的行和列之间建立连接,在该行和列之间放置开关。
要读取按键,我们需要将行配置为输出和列作为输入。
在向行应用信号之后读取列以确定是否按下了键,如果按下了按键,则按下哪个键。
例
在这里,我们将使用AT89S52(8051)连接4×4键盘,并在LCD16x2上显示按下的键。
电路连接原理图
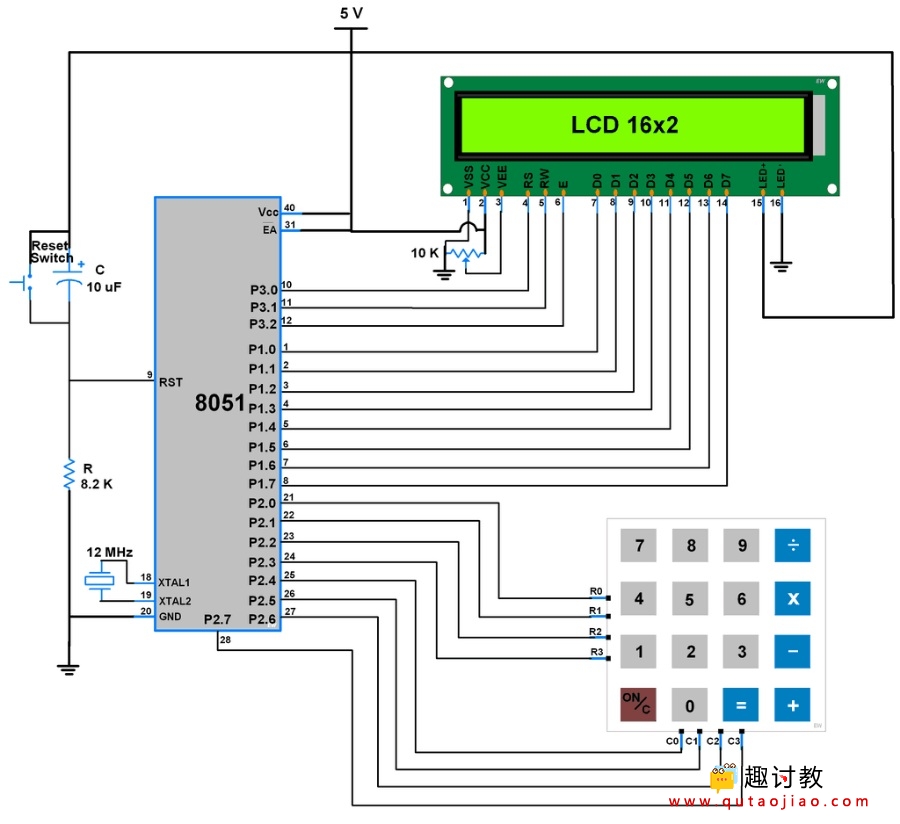
键盘与8051连接
程序
/*
4x4 Keypad Interfacing with 8051(AT89s52)
https://www.qutaojiao.com
*/
#include
#include
#include
#include "LCD_8_bit.h" /* 16x2 LCD header file*/
#define keyport P1
sbit RS = P3^5; /* RS(register select) for LCD16x2 */
sbit RW = P3^6; /* RW(Read/write) for LCD16x2 */
sbit ENABLE = P3^7; /* EN(Enable) pin for LCD16x2*/
unsigned char keypad[4][4] = {{'7','8','9','/'},
{'4','5','6','x'},
{'1','2','3','-'},
{' ','0','=','+'} };
unsigned char colloc, rowloc;
unsigned char key_detect()
{
keyport=0xF0; /*set port direction as input-output*/
do
{
keyport = 0xF0;
colloc = keyport;
colloc&= 0xF0; /* mask port for column read only */
}while(colloc != 0xF0); /* read status of column */
do
{
do
{
delay(20); /* 20ms key debounce time */
colloc = (keyport & 0xF0); /* read status of column */
}while(colloc == 0xF0); /* check for any key press */
delay(1);
colloc = (keyport & 0xF0);
}while(colloc == 0xF0);
while(1)
{
/* now check for rows */
keyport= 0xFE; /* check for pressed key in 1st row */
colloc = (keyport & 0xF0);
if(colloc != 0xF0)
{
rowloc = 0;
break;
}
keyport = 0xFD; /* check for pressed key in 2nd row */
colloc = (keyport & 0xF0);
if(colloc != 0xF0)
{
rowloc = 1;
break;
}
keyport = 0xFB; /* check for pressed key in 3rd row */
colloc = (keyport & 0xF0);
if(colloc != 0xF0)
{
rowloc = 2;
break;
}
keyport = 0xF7; /* check for pressed key in 4th row */
colloc = (keyport & 0xF0);
if(colloc != 0xF0)
{
rowloc = 3;
break;
}
}
if(colloc == 0xE0)
{
return(keypad[rowloc][0]);
}
else if(colloc == 0xD0)
{
return(keypad[rowloc][1]);
}
else if(colloc == 0xB0)
{
return(keypad[rowloc][2]);
}
else
{
return(keypad[rowloc][3]);
}
}
int main(void)
{
LCD_Init();
LCD_String_xy(1,0,"Press a key");
while(1){
LCD_Command(0xc0);
LCD_Char(key_detect()); /* Display which key is pressed */
}
}
本教程完整工程项目下载: