目录
介绍
LCD(液晶显示器)用于显示嵌入式系统中的状态或参数。
LCD 16×2是16引脚器件,具有8个数据引脚(D0-D7)和3个控制引脚(RS,RW,EN)。其余5个引脚用于LCD的电源和背光。
控制引脚可帮助我们在命令模式或数据模式下配置LCD。它们还有助于配置读取模式或写入模式以及何时进行读取或写入。
LCD 16×2可根据应用要求以4位模式或8位模式使用。为了使用它,我们需要在命令模式下向LCD发送某些命令,一旦根据我们的需要配置LCD,我们就可以在数据模式下发送所需的数据。
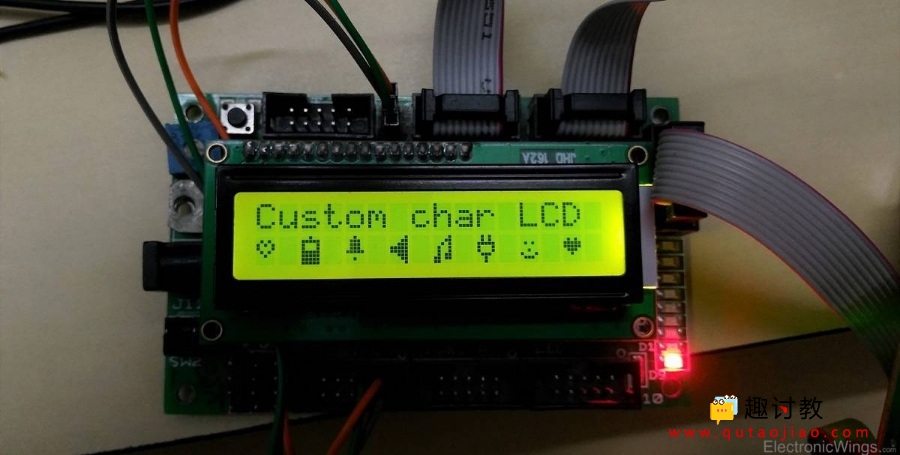
LCD 16×2自定义字符
接口图
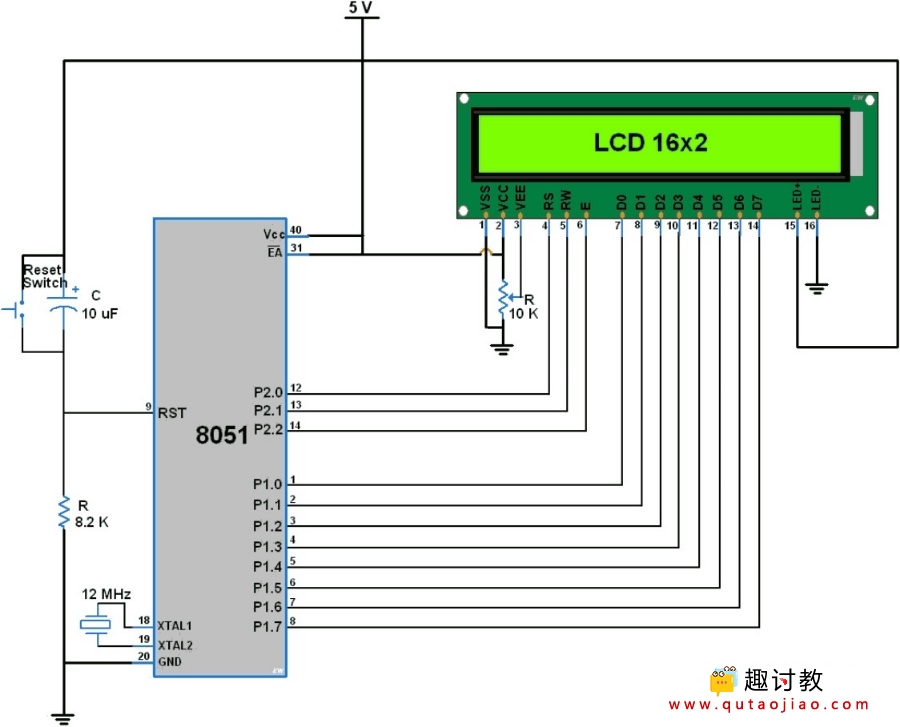
LCD16x2与8051接口
假设,我们决定将“Bell”形状自定义字符放在模式编号1,然后将它们存储在CGRAM中使用以下函数。
void LCD_Custom_Char (unsigned char loc, unsigned char *msg)
{
unsigned char i;
if(loc<8)
{
/* Command 0x40 and onwards forces the device to point CGRAM address */
LCD_Command (0x40 + (loc*8));
for(i=0;i<8;i++) /* Write 8 byte for generation of 1 character */
LCD_Char(msg[i]);
}
}
上面的函数将用于在CGRAM中存储自定义字符。
显示自定义字符
在CGRAM中存储所有自定义字符后,我们可以在LCD16x2上显示它。
要显示自定义字符,只需将自定义字符编号(从0到7)作为数据提供给LCD16x2。
例1
程序在LCD16x2上显示不同的自定义字符。
程序:
/*
LCD16x2 8 bit 8051 custom character
https://www.qutaojiao.com
*/
#include
sfr lcd_data_port=0x90; /* P1 port as data port */
sbit rs=P2^0; /* Register select pin */
sbit rw=P2^1; /* Read/Write pin */
sbit en=P2^2; /* Enable pin */
void delay(unsigned int count) /* Function to provide delay Approx 1ms */
{
int i,j;
for(i=0;ifor(j=0;j<112;j++);
}
void LCD_Command (char cmd) /* LCD16x2 command funtion */
{
lcd_data_port= cmd;
rs=0; /* command reg. */
rw=0; /* Write operation */
en=1;
delay(1);
en=0;
delay(5);
}
void LCD_Char (char char_data) /* LCD data write function */
{
lcd_data_port=char_data;
rs=1; /*Data reg.*/
rw=0; /*Write operation*/
en=1;
delay(1);
en=0;
delay(5);
}
void LCD_String (char *str) /* Send string to LCD function */
{
int i;
for(i=0;str[i]!=0;i++) /* Send each char of string till the NULL */
{
LCD_Char (str[i]); /* Call LCD data write */
}
}
void LCD_String_xy (char row, char pos, char *str) /* Send string to LCD function */
{
if (row == 0)
LCD_Command((pos & 0x0F)|0x80);
else if (row == 1)
LCD_Command((pos & 0x0F)|0xC0);
LCD_String(str); /* Call LCD string function */
}
void LCD_Init (void) /* LCD Initialize function */
{
delay(20); /* LCD Power ON Initialization time >15ms */
LCD_Command (0x38); /* Initialization of 16X2 LCD in 8bit mode */
LCD_Command (0x0C); /* Display ON Cursor OFF */
LCD_Command (0x06); /* Auto Increment cursor */
LCD_Command (0x01); /* clear display */
LCD_Command (0x80); /* cursor at home position */
}
void LCD_Custom_Char (unsigned char loc, unsigned char *msg)
{
unsigned char i;
if(loc<8)
{
/* Command 0x40 and onwards forces the device to point CGRAM address */
LCD_Command (0x40 + (loc*8));
for(i=0;i<8;i++) /* Write 8 byte for generation of 1 character */
LCD_Char(msg[i]);
}
}
余下程序:
例2
动画:豆子追逐点
/*
LCD16x2 8 bit 8051 animation
https://www.qutaojiao.com
*/
#include
sfr lcd_data_port=0x90; /* P1 port as data port */
sbit rs=P2^0; /* Register select pin */
sbit rw=P2^1; /* Read/Write pin */
sbit en=P2^2; /* Enable pin */
void lcd_built(void);
unsigned char addr,i;
void delay(unsigned int count) /* Function to provide delay Approx 1ms */
{
int i,j;
for(i=0;ifor(j=0;j<112;j++);
}
void LCD_Command (char cmd) /* LCD16x2 command funtion */
{
lcd_data_port= cmd;
rs=0; /* command reg. */
rw=0; /* Write operation */
en=1;
delay(1);
en=0;
delay(5);
}
void LCD_Char (char char_data) /* LCD data write function */
{
lcd_data_port=char_data;
rs=1; /*Data reg.*/
rw=0; /*Write operation*/
en=1;
delay(1);
en=0;
delay(5);
}
void LCD_String (char *str) /* Send string to LCD function */
{
int i;
for(i=0;str[i]!=0;i++) /* Send each char of string till the NULL */
{
LCD_Char (str[i]); /* Call LCD data write */
}
}
void LCD_String_xy (char row, char pos, char *str) /* Send string to LCD function */
{
if (row == 0)
LCD_Command((pos & 0x0F)|0x80);
else if (row == 1)
LCD_Command((pos & 0x0F)|0xC0);
LCD_String(str); /* Call LCD string function */
}
void LCD_Init (void) /* LCD Initialize function */
{
delay(20); /* LCD Power ON Initialization time >15ms */
LCD_Command (0x38); /* Initialization of 16X2 LCD in 8bit mode */
LCD_Command (0x0C); /* Display ON Cursor OFF */
LCD_Command (0x06); /* Auto Increment cursor */
LCD_Command (0x01); /* Clear display */
LCD_Command (0x80); /* Cursor at home position */
}
void LCD_Clear()
{
LCD_Command (0x01); /* Clear display */
LCD_Command (0x80); /* Cursor at home position */
}
void LCD_Custom_Char (unsigned char loc, unsigned char *msg)
{
unsigned char i;
if(loc<8)
{
LCD_Command (0x40 + (loc*8));
for(i=0;i<8;i++)
LCD_Char(msg[i]);
}
}
void show_set1()
{
LCD_Char(0x0);
LCD_Char(0x01);
}
void show_set2()
{
LCD_Char(0x2);
LCD_Char(0x3);
}
void show_dots(char j)
{
LCD_Command(0x80|(addr&0x0f));
LCD_Char(j);
}
int main(void)
{
LCD_Init();
LCD_String("Ewings");
LCD_Command(0x0c3);
LCD_String("Animation");
delay(300);
lcd_built();
addr=0xc1;
while(1)
{
addr=0xc1;
i=4;
/* showing set 1 left to right rolling */
do{
LCD_Command(addr++);
show_set1();
show_dots(i);
if(i<7)
i++;
else
i=4;
delay(200);
LCD_Clear();
LCD_Command(addr++);
show_set2();
show_dots(i);
if(i<7)
i++;
else
i=7;
delay(200);
LCD_Clear();
}while(addr<0xce);
/* showing set 2 right to left rolling */
do{
LCD_Command(addr--);
show_set1();
show_dots(i);
if(i<7)
i++;
else
i=4;
delay(200);
LCD_Clear();
LCD_Command(addr--);
show_set2();
show_dots(i);
if(i<7)
i++;
else
i=7;
delay(200);
LCD_Clear();
}while(addr>0xc2);
}
}
余下程序:
LCD1602字模取码汉字取模软件下载:
工程项目下载:
可爱 ✗笑哭了✗ ✗笑哭了✗ ✗笑哭了✗ ✗笑哭了✗
嘿嘿